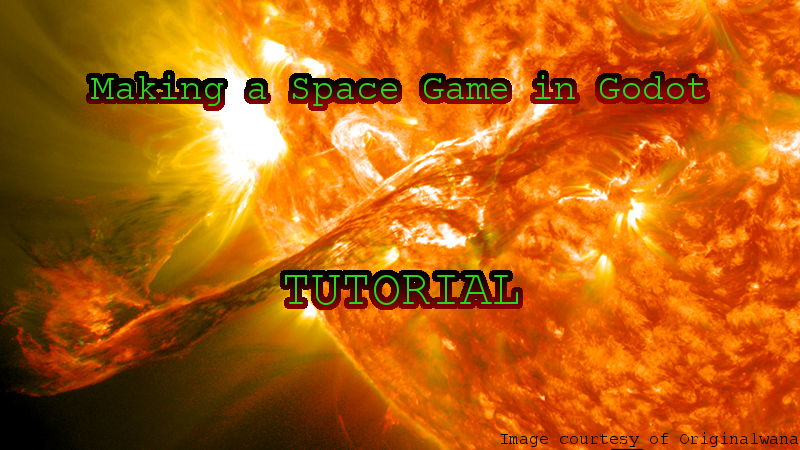
Making a Game - Bug Fixes and Server Build - EP 24
by Damien
Video Tutorial
Aims of this Tutorial
- Show the current health and energy on GUI.
- Fix the laser length bug.
- Make a build that can run on a server.
Showing Health and Energy
One thing that we failed to do was to transfer the final energy to the client for calculations like we did with the health, so we just need to copy that code for the energy. We can then show this energy by using the scale factors that we figured out previously for the battery icon. We can then call this function in the process method. We can also use a similar method for the health bar.
onready var health_bar : ColorRect = $StatusPanel/HealthBarContainer/HealthBar
func _update_battery_visuals(value : float) -> void:
$WeaponsPanel/FillingBlocker.margin_bottom = 65 - (clamp(value, 0.0, 100.0) * 1.2)
func _update_health_bar(value : float) -> void:
health_bar.anchor_left = 0.5 - clamp(value, 0.0, 100.0)/200
health_bar.anchor_right = 0.5 + clamp(value, 0.0, 100.0)/200
The health bar is made of a panel, a colour rectangle (black) and another colour rectangle on top of that which we modify. The code here will squish the bar down symmetrically in the x axis based on the input health. We are clamping the value here to avoid the bar from under/overflowing the box, which would be bad.
Fixing the Laser Length Bug
This is actually a very simple fix. Since the CSGCylinder is a child of the Raycast which is a child of the gun visuals, which has a scale of 0.1 in all axes, the cylinder was made 10 times shorter than intended. The fix is to simply multiply the length of the laser by 10!
func _update_laser(length : float) -> void:
$GunContainer/RayCast/CSGCylinder.height = length*10
$GunContainer/RayCast/CSGCylinder.translation = Vector3(0, length*5, 0)
Creating a Server Build
Most servers run some distribution of Linux and do not have an X server. This means that there are no preset exports we can use to run this on a server. The solution is to select a Linux export and only export the .pck
file. This cannot run by itself, but can be used by the Godot engine. You can use a server build of Godot to run this without having an editor. Once you have exported your file you send it to your server with the following command.
scp <Your game>.pck <username>@<Your server>:<remote directory>
You can then ssh into your server, create a new user to run the game (do not run your game as root in case there are critical security vulnerabilities) with useradd -m <username>
. Then copy the pck into their home folder (/home/<username>). You can then switch to this new user with su <username>
and download the corresponding server build for the build you were using to make the pkg. For example, I am on 3.2.1 so I run the following command:
wget https://downloads.tuxfamily.org/godotengine/3.2.1/Godot_v3.2.1-stable_linux_server.64.zip
Once the download is complete, you can extract it by running unzip Godot_v3.2.1-stable_linux_server.64.zip
. You can then run the server by running ./Godot_v3.2.1-stable_linux_server.64 --main-pck <Your game>.pck
. In a later episode, we might wrap this in a systemd unit file, but for now, we can keep it as is.