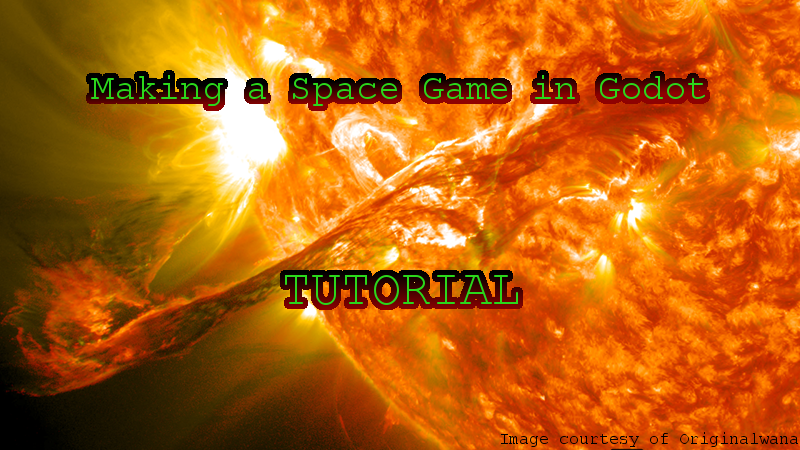
Making a Game - Incremental Turns - EP 19
by Damien
Video Tutorial
Aims of this Tutorial
- Be able to calculate the last five seconds of the plan on both the client and the server.
- Use this to shorten the time taken to calculate the plan on the server, thus decreasing the wait between turns.
Getting the last state of the players
This will be different for the client and the server. The server will get this information straight from the calculation and will send it to the clients. This way, we know that everyone will be on the same page. Therefore, we need to add the following to the send_all_plans()
function:
end_translation = self.translation
end_rotation = self.rotation
end_linear_velocity = self.linear_velocity
end_rotational_velocity = self.angular_velocity
rpc("r_end_turn", time_of_death, end_translation, end_rotation, end_linear_velocity, end_rotational_velocity)
As you can see the rpc call has been modified, so we need to make a similar change on the client, so modify the function to include:
Calculating the last 5 seconds
This is largely the same on the server and client, but there are some subtleties that we will cover. We will show server code and mention the modifications required. We need a function similar to play_all_plans()
to run the last 5 seconds.
The major changes are that we set our initial state based on where we ended up last turn and that we check if died in the previous turn to find out if we need to set ourselves to alive. On the client, we would get rid of the entire if statement. has_died
is a bool that we set to true the first time a player dies and turn_number
is an int that we increase by one each time we send our plans!
We also need to make a small modification to the calculate_turns()
function, since we need to still use the full plan on turn 0, but every other turn, we can use the last plan!
func calculate_plans():
if turn_number == 0:
play_full_plan()
else:
play_last_plan()
waiting_for_completion = true
Finally, only on the client, we need to hook up a new 'play last turn' button to a function similar to the one above, just without setting waiting_for_completion
at the end.