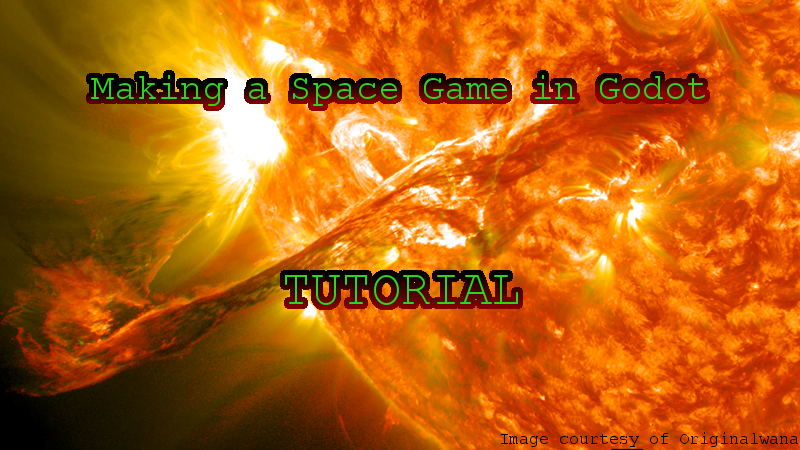
Making a Game - Visualising the New Weapons - EP 29
by Damien
Video Tutorial
Aims of this Tutorial
- Create a system for showing the firing arc we created last episode.
- Learn how to more effectively use Git and Gitea in order for your commits to work well when other people get involved.
- Update the current music system to allow multiple different tracks to be set for one scenario, and one is picked at random.
Showing the Firing Arc
We already have the mesh for the collider, so if we drag this into our player scene, set it as the child of the collision shape for the cone and reset its transform, it should perfectly overlay the collision shape. This is because it inherits the transform of the collision shape, thus multiplying by the identity matrix does nothing and we have the same overall transform. If we then apply the glass material we used for the arrows, we have a cool looking glass cone!
We now need to create an input method for deciding which cones to show. The solution to this is to create a new node under the weapons panel. Since we want to offer the player a few options for who should show their cone, we want to use an option menu. To edit the available choices, select the menu in the scene, and at the top of the viewport, there should be an option called "Items". Select that and add in 3 different options: "None", "Self" and "Everyone". You can ignore all other options apart from the label which should be set to these values respectively. Now you have a working option menu, we need to hook this up to the script.
To do this, connect the item_selected
signal to the player script and call it what you want. Now, we can create a function in the player script to change the visibility of their cone.
func set_firing_arc_visibility(toggle : bool) -> void:
$GunContainer/Cone/CollisionShape/Cone.visible = toggle
However, we also need to be able to change the visibility of all players' cones. To make this easy, we can tell the arena to do this for us. This is very similar to the function on the arena that tells all the players to run their plans.
func set_all_firing_arc_visibilities(toggle : bool) -> void:
for player in players.values():
player.set_firing_arc_visibility(toggle)
Finally, we can create the body for the function that was created by making the signal. There are 3 different options that can be selected, so we need 3 different outcomes. One is that we turn everyone's cone off, one is that we turn everyone's cone on, and the last one is that we turn ours on but everyone else's off. In this last case, it is simpler to just turn everyone's cone off, and then turn ours on afterwards, to make use of the functions we have already made.
func _on_WeaponVisualiserMenu_item_selected(index):
if index == 0:
get_parent().set_all_firing_arc_visibilities(false)
elif index == 1:
get_parent().set_all_firing_arc_visibilities(false)
set_firing_arc_visibility(true)
elif index == 2:
get_parent().set_all_firing_arc_visibilities(true)
The complete changes made can be found in this commit on my Gitea.
Pushing these Changes Publicly
Now that you have made some changes, you want these to be accepted into the public code base. To do this, you need to create a pull request. While these instructions are for Gitea, they will work for GitHub, GitLab and most other popular git web clients.
Firstly, we need to follow the standard git procedure of adding files to be staged using git add *
and committing them with a useful message using git commit -m "Useful message"
. Now we differ here. If you do not have write permission to the repository, you need to fork it first. There is a button in the top right that says fork. If you are logged in, clicking this button will fork the repository and you will have one under your account. Now you can push your changes there (make sure to update your upstream url in git with git remote set-url origin <url>
!). If you have write permissions, in order keep organised, push to a different, new branch using git push origin master:new_branch
.
Now, both methods converge again. In your fork/branch, a new button should appear called "New Pull Request". Clicking this button should automatically create the pull request correctly, but you can double check and change where it is merging to and where it is pulling from. Once you are happy that everything is correct, you can press the create pull request button. Now you can write a more detailed message saying what your pull request does and any details that might be important for the maintainers to know. Some projects might have pull request templates set up to guide you along this process. Once you are done with this, you can add the relevant labels, add the participants and click the create button.
If you have write access to the repository, you now need to merge this PR to get the code into master. If you don't have write access, you need to wait on the maintainers for feedback or, if they are happy with it, for them to merge it. To merge a PR, open the PR and, if you have write access and there are no conflicts, there should be a large purple button to merge the request. Clicking this will cause Gitea to merge the code into master for you. After this, the new branch is useless and may be deleted with impunity (there is a button on the merged PR as a shortcut for you).
Modifying the Music Player
Since we want to choose a song randomly, we need to change the music dictionary to have a list of potential themes to use as the values. Then we need to update the switch theme function to pick a random song from the options. This can be done by shuffling the list and picking the first option. Just remember to randomise the random number generator first.
func switch_theme(name : String) -> void:
if name in music_dict.keys():
var music_list = music_dict[name]
music_list.shuffle()
stream = music_list[0]
current_music_array = name
else:
get_parent().console_print("Could not find music array of name, " + name)
playing = true
Since we want to pick a different theme each time, we store the current key in current_music_array
so that when the track finished, the is_finished
signal, which is self connected, calls switch_theme
again with this name to start playing a new song from the list.
func _on_finished():
switch_theme(current_music_array)
The complete changes for this are found in this commit on my Gitea.